Smart Environmental Monitoring System Or Weather Station

The Smart Environmental Monitoring System utilizes a combination of temperature, light, and moisture sensors to provide real-time data on environmental conditions. The project employs an Arduino board interfaced with sensors to measure temperature, light intensity, and soil moisture levels. The data collected is displayed on an LCD screen and can be transmitted to a computer for further analysis.
The circuit comprises Arduino, Adafruit_LiquidCrystal, and various sensors. Temperature is measured using a thermal sensor (TERMAL_INPIN), light intensity using a light sensor (LIGHT_INPIN), and soil moisture with a moisture sensor (MOISTURE_INPIN). The Adafruit_LiquidCrystal module is used for the LCD display.
Component List:
Name | Quantity | Component |
---|---|---|
SEN1 | 1 | Soil Moisture Sensor |
U1 | 1 | Arduino Uno R3 |
U2 | 1 | Temperature Sensor [TMP36] |
Q1 | 1 | Ambient Light Sensor [Phototransistor] |
R1 | 1 | 1 kΩ Resistor |
U3 | 1 | MCP23008-based, 32 LCD 16 x 2 (I2C) |
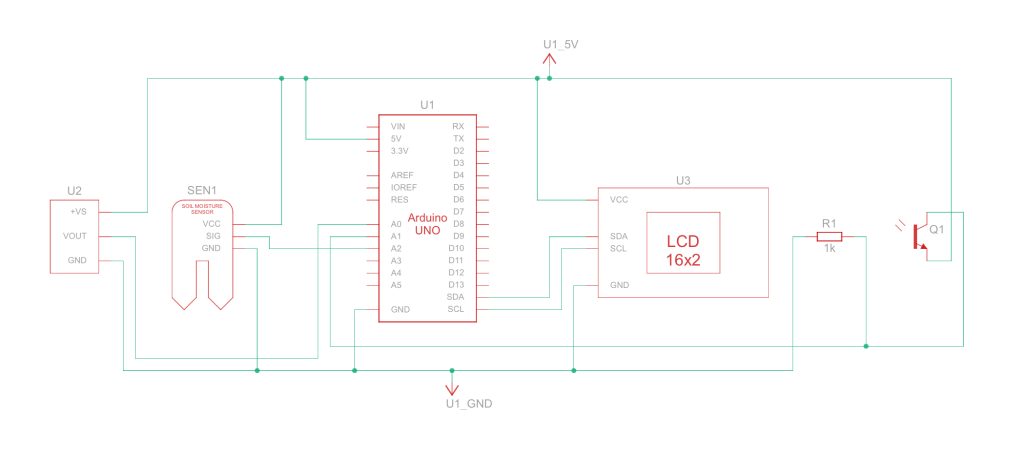
Arduino Code
#include <Adafruit_LiquidCrystal.h>
#define TERMAL_INPIN A0
#define LIGHT_INPIN A1
#define MOISTURE_INPIN A2
Adafruit_LiquidCrystal lcd_out = Adafruit_LiquidCrystal(0);
class Sensors {
public:
int temperature, light, moisture;
inline static const char* names[] = {"Temperature", "Light", "Moisture"};
inline static const char* units[] = {" C", "%", "%"}; inline static const char lname[] = {"T:", "L:", "M:"};
Sensors() {
this->temperature = 0;
this->light = 0;
this->moisture = 0;
}
void take() {
this->temperature = map(analogRead(TERMAL_INPIN), 20, 358, -40, 125);
this->light = map(analogRead(LIGHT_INPIN), 0, 13, 0, 100);
this->moisture = map(analogRead(MOISTURE_INPIN), 0, 876, 0, 100);
}
void genData(int buffer[3]) {
int data[] = {this->temperature, this->light, this->moisture};
for (int i = 0; i < 3; i++) buffer[i] = data[i];
}
void print_lcd(void) {
int data[3];
this->genData(data);
lcd_out.setCursor(0, 0);
for (int i = 0; i < 3; i++) { if (i == 2) lcd_out.setCursor(0, 1); lcd_out.print(this->lname[i]);
delay(100);
lcd_out.print(data[i]);
delay(100);
lcd_out.print(" ");
delay(100);
}
}
void print_serial(void) {
int data[3];
this->genData(data);
for (int i = 0; i < 3; i++) { Serial.print("[INFO] "); Serial.print(this->names[i]);
Serial.print(": ");
Serial.print(data[i]);
Serial.println(this->units[i]);
}
Serial.println("");
}
};
void copy(Sensors& dest, Sensors& src);
Sensors now_sen = Sensors();
Sensors prev_sen = Sensors();
void setup() {
Serial.begin(9600);
lcd_out.begin(16, 2);
Serial.println("92100588034");
}
void loop() {
now_sen.take();
if (prev_sen.moisture > 99 && now_sen.moisture < 100)
lcd_out.clear();
now_sen.print_lcd();
// now_sen.print_serial();
copy(prev_sen, now_sen);
delay(1000);
}
void copy(Sensors& dest, Sensors& src) {
dest.temperature = src.temperature;
dest.light = src.light;
dest.moisture = src.moisture;
}
This project offers a cost-effective and efficient solution for monitoring environmental parameters in agriculture or home settings. The LCD provides immediate feedback, and the system can be expanded for remote monitoring through wireless communication.
By understanding and responding to changes in temperature, light, and soil moisture, users can make informed decisions to optimize conditions for plant growth or maintain a comfortable indoor environment. The Smart Environmental Monitoring System is a valuable tool for enhancing resource efficiency and supporting sustainable practices.