Arduino Calculator with Keypad and LCD Display
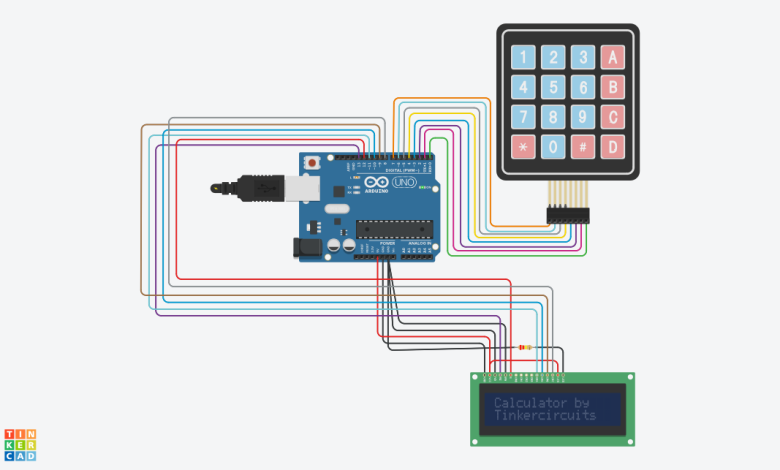
The Arduino Calculator project combines hardware components with programming to create a functional calculator. Using a 4×4 keypad for input and a 16×2 LCD for output, the device allows users to perform basic arithmetic operations.
The system accumulates numeric inputs until an operator is pressed, at which point it computes and displays the result on the LCD. The ‘C’ key resets the calculator, providing a user-friendly interface for quick calculations
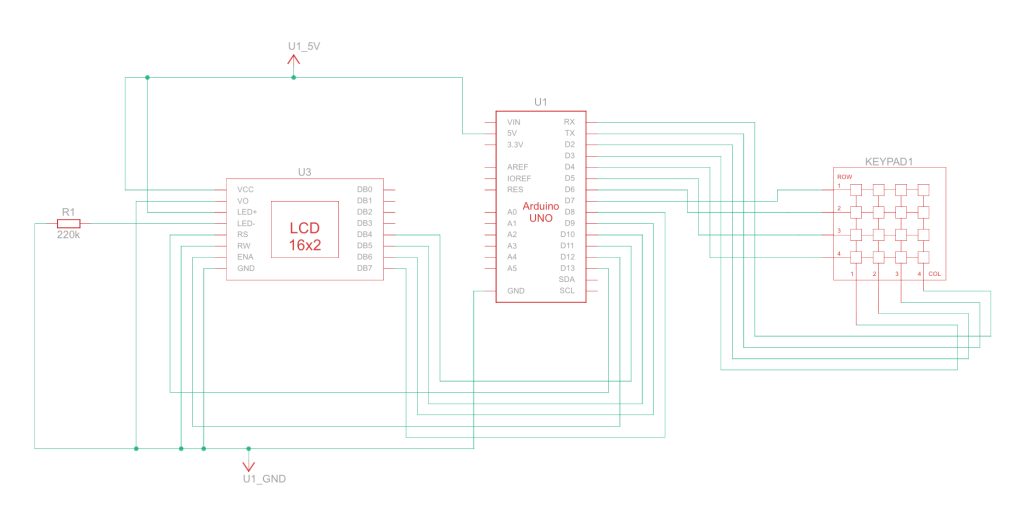
The circuit involves connecting a 4×4 keypad and a 16×2 LCD to an Arduino board. The keypad’s rows and columns are connected to specific digital pins on the Arduino, enabling the detection of key presses. The LCD is interfaced through its data pins, allowing the Arduino to send and receive information from the display.
Component List:
Name | Quantity | Component |
---|---|---|
U1 | 1 | Arduino Uno R3 |
U3 | 1 | LCD 16 x 2 |
KEYPAD1 | 1 | Keypad 4×4 |
R1 | 1 | 220 kΩ Resistor |
This Arduino Calculator project offers a hands-on experience in combining input and output devices, making it an excellent educational tool. Users can explore the synergy between hardware and software, gaining insights into keypad interfacing, LCD displays, and basic programming logic for arithmetic operations.
The project’s simplicity makes it accessible for beginners, yet it showcases the fundamental principles of microcontroller-based systems. Overall, it serves as a practical introduction to Arduino-based applications and encourages further exploration of interactive electronics projects.
Arduino Code:
#include <Keypad.h>
#include <Wire.h>
#include <LiquidCrystal.h>
LiquidCrystal lcd(13, 12, 11, 10, 9, 8);
long first = 0;
long second = 0;
double total = 0;
char customKey;
const byte ROWS = 4;
const byte COLS = 4;
char keys[ROWS][COLS] = {
{'1','2','3','+'},
{'4','5','6','-'},
{'7','8','9','*'},
{'C','0','=','/'}
};
byte rowPins[ROWS] = {7,6,5,4};
byte colPins[COLS] = {3,2,1,0};
Keypad customKeypad = Keypad( makeKeymap(keys), rowPins, colPins, ROWS, COLS);
void setup() {
lcd.begin(16, 2);
for (int i = 0; i <= 3; i++);
lcd.setCursor(0,0);
lcd.print("Calculator by");
lcd.setCursor(0,1);
lcd.print("Tinkercircuits");
delay(4000);
lcd.clear();
lcd.setCursor(0, 0);
}
void loop() {
customKey = customKeypad.getKey();
switch(customKey) {
case '0' … '9':
lcd.setCursor(0,0);
first = first * 10 + (customKey - '0');
lcd.print(first);
break;
case '+': first = (total != 0 ? total : first);
lcd.setCursor(0,1);
lcd.print("+");
second = SecondNumber();
total = first + second;
lcd.setCursor(0,3);
lcd.print(total);
first = 0, second = 0;
break;
case '-': first = (total != 0 ? total : first);
lcd.setCursor(0,1);
lcd.print("-");
second = SecondNumber();
total = first - second;
lcd.setCursor(0,3);
lcd.print(total);
first = 0, second = 0;
break;
case '*': first = (total != 0 ? total : first);
lcd.setCursor(0,1);
lcd.print("*");
second = SecondNumber();
total = first * second;
lcd.setCursor(0,3);
lcd.print(total);
first = 0, second = 0;
break;
case '/': first = (total != 0 ? total : first);
lcd.setCursor(0,1);
lcd.print("/");
second = SecondNumber();
lcd.setCursor(0,3);
second == 0 ? lcd.print("Invalid") : total = (float)first / (float)second;
lcd.print(total);
first = 0, second = 0;
break;
case 'C': total = 0;
lcd.clear(); break;
}
}
long SecondNumber() {
while( 1 ) {
customKey = customKeypad.getKey();
if(customKey >= '0' && customKey <= '9') {
second = second * 10 + (customKey - '0');
lcd.setCursor(0,2);
lcd.print(second);
}
if(customKey == '=') break;
}
return second;
}