GPS Data Display with Arduino and LCD

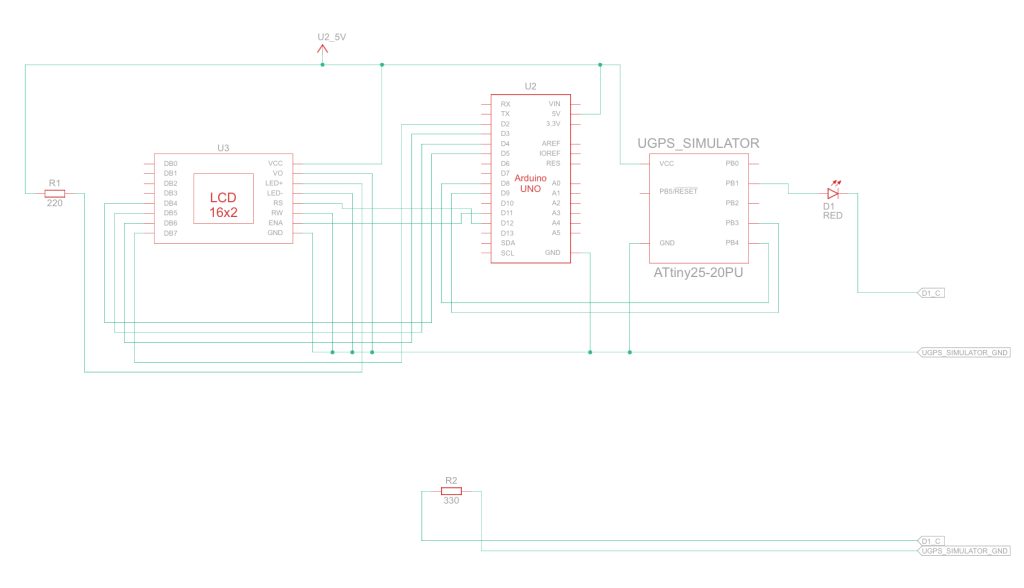
This project utilizes an Arduino, a GPS module, and an LCD display to receive and showcase real-time GPS data. The Arduino is programmed to communicate with the GPS module, extract essential information like latitude, longitude, and velocity, and then display it on the LCD screen.
The GPS module communicates with the Arduino through the SoftwareSerial library, while the LiquidCrystal library manages the LCD.
Component List:
Name | Quantity | Component |
---|---|---|
U2 | 1 | Arduino Uno R3 |
U3 | 1 | LCD 16 x 2 |
R1 | 1 | 220 Ω Resistor |
UGPS_simulator | 1 | ATtiny |
R2 | 1 | 330 Ω Resistor |
D1 | 1 | Red LED |
The hardware setup involves connecting the LCD to specific digital pins on the Arduino and establishing a serial communication link with the GPS module. Additionally, SoftwareSerial is employed to create a secondary serial connection to the GPS module.
Arduino Pins:
- LCD RS pin to digital pin 12
- LCD Enable pin to digital pin 11
- LCD D4-D7 pins to digital pins 5-2
- GPS module connected to SoftwareSerial pins 8 (RX) and 9 (TX)
Arduino Code:
#include <SoftwareSerial.h>
#include <LiquidCrystal.h>
LiquidCrystal lcd(12, 11, 5, 4, 3, 2);
SoftwareSerial portTwo(8, 9); // RX=8, TX=9
char inByte;
void setup() {
lcd.begin(16, 2);
lcd.setCursor(0, 0);
lcd.print("GPS data");
Serial.begin(9600);
Serial.println("Test PC connection");
portTwo.begin(9600);
}
void loop() {
portTwo.listen();
if (portTwo.available() > 0) {
Serial.println("Data from GPS:");
inByte = portTwo.read();
Serial.write(inByte);
FSM(inByte);
}
delay(1000);
}
void FSM(char inByte) {
char LAT = 0x50;
char LOG = 0x20;
char VEL = 0X30;
LCD_print(LAT, LOG, VEL);
}
void LCD_print(char LAT, char LOG, char VEL) {
lcd.setCursor(0, 0);
lcd.print("Lat Long Vel");
lcd.setCursor(0, 1);
lcd.print(LAT, HEX);
lcd.setCursor(2, 1);
lcd.print('N');
lcd.setCursor(4, 1);
lcd.print(LOG, HEX);
lcd.setCursor(7, 1);
lcd.print('E');
lcd.setCursor(9, 1);
lcd.print(VEL, HEX);
lcd.setCursor(12, 1);
lcd.print("KM/h");
}
This project creates a simple and effective GPS data display system using readily available components. It showcases the integration of software libraries for serial communication and LCD operation.
Such projects could be extended for navigation systems, location-based reminders, or any application requiring real-time GPS data visualization.
The flexibility of Arduino and the simplicity of the circuit make it accessible for hobbyists and students interested in exploring GPS technology.