Electronic Voting Machine with Arduino
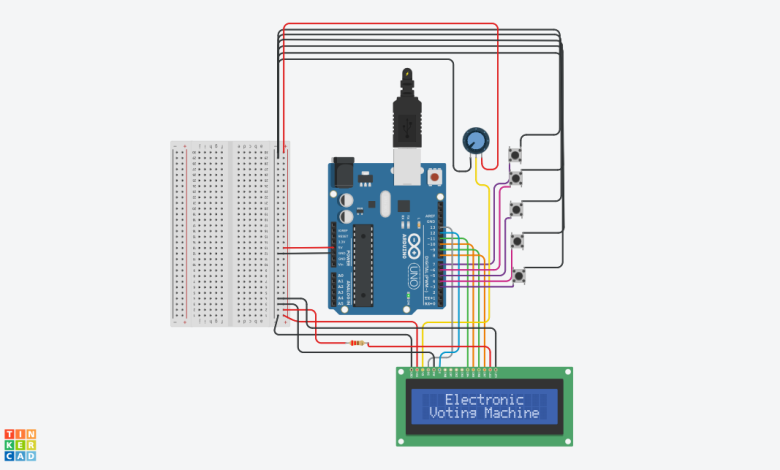
In this project we will see how to make Electronic Voting Machine with Arduino.

The Electronic Voting Machine (EVM) with Arduino is a project that brings modern technology to the voting process. Using Arduino and a LiquidCrystal display, this EVM is designed to handle multiple candidates and provide real-time vote counts.
The system includes push buttons (S1-S5) for each candidate, and voters can cast their votes with a simple button press.
The Arduino microcontroller processes the inputs and updates the display to show the current vote counts for each candidate. The system also declares the winner based on the highest vote count.
Component List:
Name | Quantity | Component |
---|---|---|
UScreen | 1 | LCD 16 x 2 |
S1 S3 S4 S5 S2 | 5 | Pushbutton |
U1 | 1 | Arduino Uno R3 |
Rpot1 | 1 | 250 kΩ Potentiometer |
R1 | 1 | 220 Ω Resistor |
The circuit consists of push buttons connected to digital input pins (S1-S5) on the Arduino and a LiquidCrystal display connected to specific digital and analog pins.
Each push button represents a candidate, and their status is read by the Arduino. The display shows the candidates and their respective vote counts. Additionally, the circuit includes resistors to ensure proper button input.
Arduino Code:
#include <LiquidCrystal.h>
LiquidCrystal lcd(13, 12, 11, 10, 9, 8);
define S1 7
define S2 6
define S3 5
define S4 4
define S5 3
int vote1 = 0;
int vote2 = 0;
int vote3 = 0;
int vote4 = 0;
void setup() {
pinMode(S1, INPUT);
pinMode(S2, INPUT);
pinMode(S3, INPUT);
pinMode(S4, INPUT);
pinMode(S5, INPUT);
lcd.begin(16, 2);
lcd.setCursor(2, 0);
lcd.print(" Electronic ");
lcd.setCursor(0, 1);
lcd.print(" Voting Machine ");
delay(3000);
digitalWrite(S1, HIGH);
digitalWrite(S2, HIGH);
digitalWrite(S3, HIGH);
digitalWrite(S4, HIGH);
digitalWrite(S5, HIGH);
lcd.clear();
lcd.setCursor(0, 0);
lcd.print("A");
lcd.setCursor(4, 0);
lcd.print("B");
lcd.setCursor(8, 0);
lcd.print("C");
lcd.setCursor(12, 0);
lcd.print("NA");
}
void loop() {
lcd.setCursor(0, 0);
lcd.print("A");
lcd.setCursor(1, 1);
lcd.print(vote1);
lcd.setCursor(4, 0);
lcd.print("B");
lcd.setCursor(5, 1);
lcd.print(vote2);
lcd.setCursor(8, 0);
lcd.print("C");
lcd.setCursor(9, 1);
lcd.print(vote3);
lcd.setCursor(12, 0);
lcd.print("NA");
lcd.setCursor(13, 1);
lcd.print(vote4);
if (digitalRead(S1) == 0)
vote1++;
while (digitalRead(S1) == 0);
if (digitalRead(S2) == 0)
vote2++;
while (digitalRead(S2) == 0);
if (digitalRead(S3) == 0)
vote3++;
while (digitalRead(S3) == 0);
if (digitalRead(S4) == 0)
vote4++;
while (digitalRead(S4) == 0);
if (digitalRead(S5) == 0) {
int vote = vote1 + vote2 + vote3 + vote4;
if (vote) {
if ((vote1 > vote2 && vote1 > vote3 && vote1 > vote4)) {
lcd.clear();
lcd.setCursor(1, 0);
lcd.print("A is Winner");
delay(3000);
lcd.clear();
} else if ((vote2 > vote1 && vote2 > vote3 && vote2 > vote4)) {
lcd.clear();
lcd.setCursor(1, 0);
lcd.print("B is Winner");
delay(3000);
lcd.clear();
} else if ((vote3 > vote1 && vote3 > vote2 && vote3 > vote4)) {
lcd.clear();
lcd.setCursor(1, 0);
lcd.print("C is Winner");
delay(3000);
lcd.clear();
} else if (vote4 > vote1 && vote4 > vote2 && vote4 > vote3) {
lcd.setCursor(0, 0);
lcd.clear();
lcd.setCursor(1, 0);
lcd.print("NA is Winner");
delay(3000);
lcd.clear();
} else {
lcd.clear();
lcd.setCursor(5, 0);
lcd.print(" TIE ");
lcd.setCursor(2, 1);
lcd.print(" No Result ");
delay(3000);
lcd.clear();
}
} else {
lcd.clear();
lcd.setCursor(2, 0);
lcd.print("No Voting…");
delay(3000);
lcd.clear();
}
vote1 = 0;
vote2 = 0;
vote3 = 0;
vote4 = 0, vote = 0;
lcd.clear();
}
}
This Electronic Voting Machine project demonstrates a practical application of Arduino in simplifying the voting process.
The circuit is user-friendly and efficient, providing a clear visual representation of votes. However, security considerations are crucial for real-world deployment to ensure the integrity of the voting system.
The project offers a foundation for further enhancements, such as incorporating biometric authentication and encryption for a more secure and reliable electronic voting solution.