Arduino-Powered Obstacle Avoiding Robot
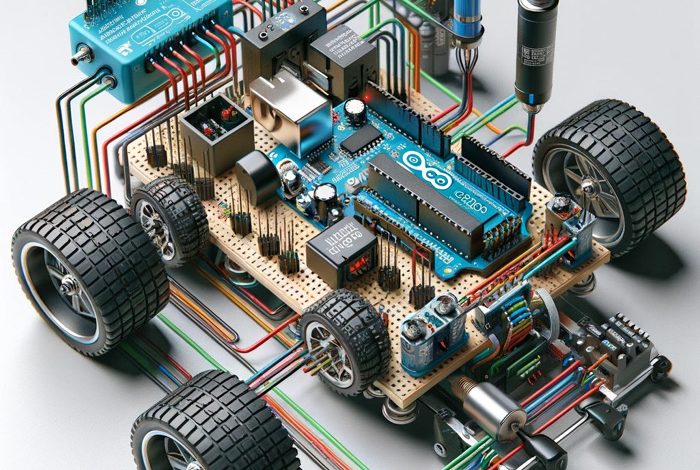
The Arduino-Powered Obstacle Avoiding Robot is an engaging and educational project that introduces robotics and automated navigation.
Utilizing an Arduino as its brain, the robot employs ultrasonic sensors to detect obstacles in its path and navigate around them. It’s a perfect project for beginners and enthusiasts interested in robotics, automation, and programming.
At the core of the project is an Arduino Uno board, which controls the robot’s movements based on the input from ultrasonic sensors like the HC-SR04. These sensors emit ultrasonic waves, and by measuring the time taken for the echo to return, the robot can calculate the distance to an obstacle and adjust its path accordingly.
This robot can be equipped with wheels and motors, enabling it to move and turn in different directions. It’s a fantastic way to learn about sensor integration, motor control, and algorithmic thinking in robotics.
The project is customizable, and additional features like line following or remote control can be added for more complexity and functionality.
Components Used:
- Arduino Uno
- HC-SR04 Ultrasonic Sensor
- Motor Driver (like L298N)
- DC Motors with Wheels
- Chassis for the Robot
- Breadboard and Connecting Wires
- Power Supply (Battery Pack)
Working Principle:
The ultrasonic sensor detects objects by emitting sound waves and measuring the time for the echo to return. The Arduino processes this information and controls the motors through the motor driver, steering the robot away from obstacles.
Circuit Diagrams:
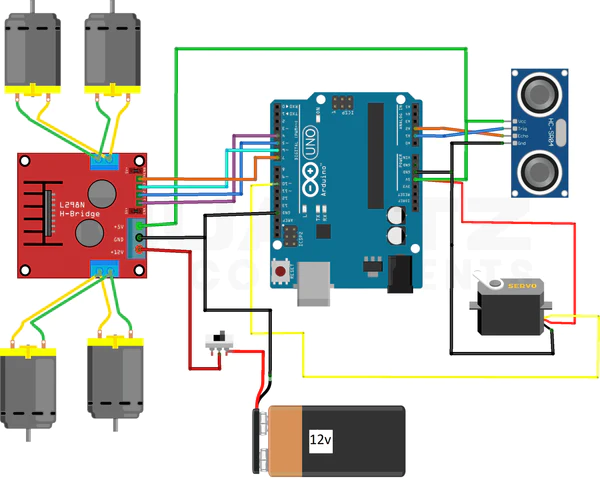
The circuit diagrams will illustrate the connections between the Arduino, ultrasonic sensor, motor driver, and the motors, showing how these components work together to enable the robot’s autonomous movement.
Arduino Code:
#include <Servo.h> //Servo motor library. This is standard library
#include <NewPing.h> //Ultrasonic sensor function library. You must install this library
//our L298N control pins
const int LeftMotorForward = 7;
const int LeftMotorBackward = 6;
const int RightMotorForward = 5;
const int RightMotorBackward = 4;
//sensor pins
#define trig_pin A1 //analog input 1
#define echo_pin A2 //analog input 2
#define maximum_distance 200
boolean goesForward = false;
int distance = 100;
NewPing sonar(trig_pin, echo_pin, maximum_distance); //sensor function
Servo servo_motor; //our servo name
void setup(){
pinMode(RightMotorForward, OUTPUT);
pinMode(LeftMotorForward, OUTPUT);
pinMode(LeftMotorBackward, OUTPUT);
pinMode(RightMotorBackward, OUTPUT);
servo_motor.attach(10); //our servo pin
servo_motor.write(115);
delay(2000);
distance = readPing();
delay(100);
distance = readPing();
delay(100);
distance = readPing();
delay(100);
distance = readPing();
delay(100);
}
void loop(){
int distanceRight = 0;
int distanceLeft = 0;
delay(50);
if (distance <= 35){
moveStop();
delay(300);
moveBackward();
delay(400);
moveStop();
delay(300);
distanceRight = lookRight();
delay(300);
distanceLeft = lookLeft();
delay(300);
if (distance >= distanceLeft){
turnRight();
moveStop();
}
else{
turnLeft();
moveStop();
}
}
else{
moveForward();
}
distance = readPing();
}
int lookRight(){
servo_motor.write(50);
delay(500);
int distance = readPing();
delay(100);
servo_motor.write(115);
return distance;
}
int lookLeft(){
servo_motor.write(170);
delay(500);
int distance = readPing();
delay(100);
servo_motor.write(115);
return distance;
delay(100);
}
int readPing(){
delay(70);
int cm = sonar.ping_cm();
if (cm==0){
cm=250;
}
return cm;
}
void moveStop(){
digitalWrite(RightMotorForward, LOW);
digitalWrite(LeftMotorForward, LOW);
digitalWrite(RightMotorBackward, LOW);
digitalWrite(LeftMotorBackward, LOW);
}
void moveForward(){
if(!goesForward){
goesForward=true;
digitalWrite(LeftMotorForward, HIGH);
digitalWrite(RightMotorForward, HIGH);
digitalWrite(LeftMotorBackward, LOW);
digitalWrite(RightMotorBackward, LOW);
}
}
void moveBackward(){
goesForward=false;
digitalWrite(LeftMotorBackward, HIGH);
digitalWrite(RightMotorBackward, HIGH);
digitalWrite(LeftMotorForward, LOW);
digitalWrite(RightMotorForward, LOW);
}
void turnRight(){
digitalWrite(LeftMotorForward, HIGH);
digitalWrite(RightMotorBackward, HIGH);
digitalWrite(LeftMotorBackward, LOW);
digitalWrite(RightMotorForward, LOW);
delay(250);
digitalWrite(LeftMotorForward, HIGH);
digitalWrite(RightMotorForward, HIGH);
digitalWrite(LeftMotorBackward, LOW);
digitalWrite(RightMotorBackward, LOW);
}
void turnLeft(){
digitalWrite(LeftMotorBackward, HIGH);
digitalWrite(RightMotorForward, HIGH);
digitalWrite(LeftMotorForward, LOW);
digitalWrite(RightMotorBackward, LOW);
delay(250);
digitalWrite(LeftMotorForward, HIGH);
digitalWrite(RightMotorForward, HIGH);
digitalWrite(LeftMotorBackward, LOW);
digitalWrite(RightMotorBackward, LOW);
}
The code for this project would involve initializing the ultrasonic sensor and motor driver, continuously reading the distance to obstacles, and commanding the motors to avoid collisions.
Thank you for reading.