Hand Gesture-Controlled Servo System
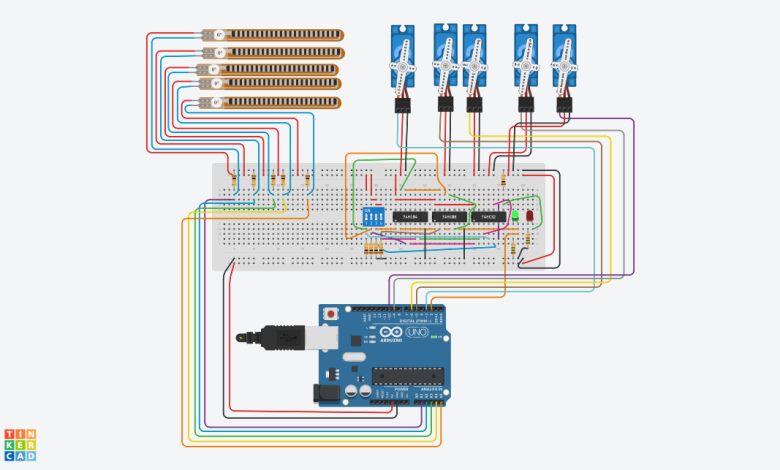
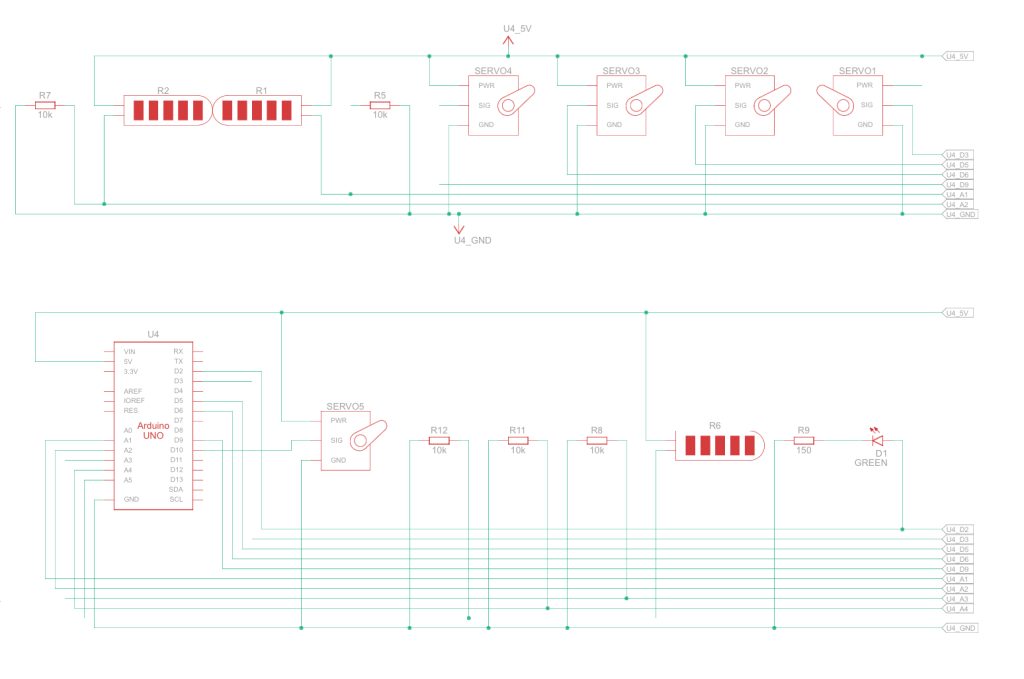
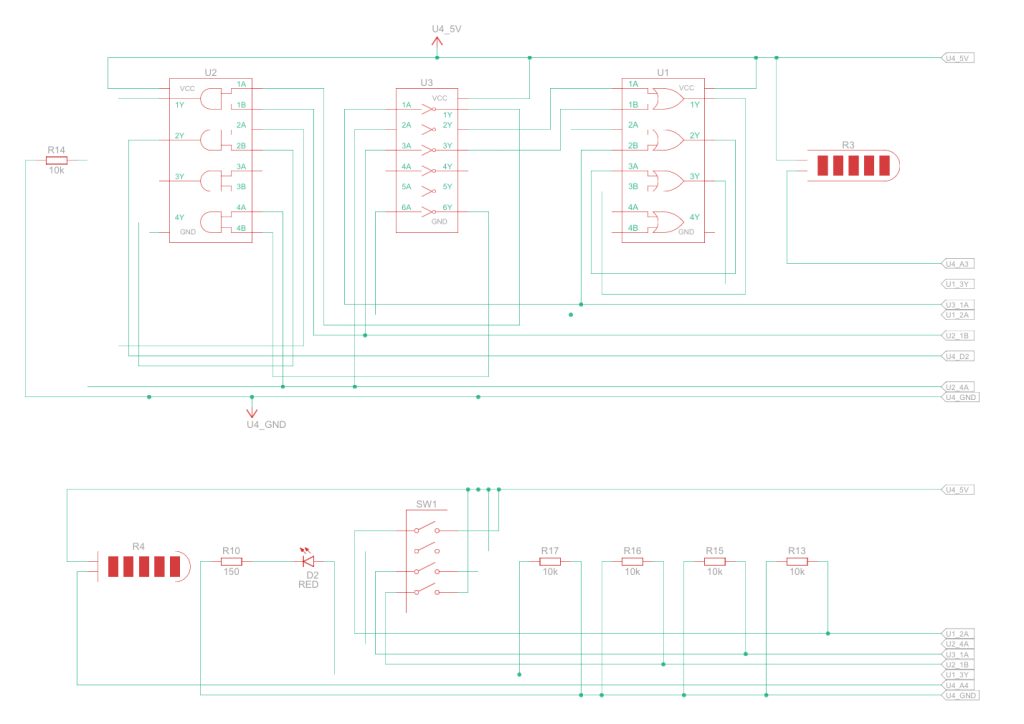
The Hand Gesture-Controlled Servo System is an interactive project that leverages flex sensors and servo motors to enable hands-free control through natural hand movements.
Five flex sensors are strategically placed on the hand to detect various degrees of flexion, translating these movements into corresponding actions performed by five servo motors.
An Arduino board processes the analog signals from the flex sensors and controls the servo motors, creating a responsive and intuitive hand gesture-controlled system.
Component List:
Name | Quantity | Component |
---|---|---|
R1 R2 R3 R4 R6 | 5 | Flex Sensor |
SERVO1 SERVO2 SERVO3 SERVO4 SERVO5 | 5 | Positional Micro Servo |
U1 | 1 | Quad OR gate |
U2 | 1 | Quad AND gate |
U3 | 1 | Hex Inverter |
R5 R7 R8 R11 R12 R13 R14 R15 R16 R17 | 10 | 10 kΩ Resistor |
U4 | 1 | Arduino Uno R3 |
SW1 | 1 | DIP Switch SPST x 4 |
D1 | 1 | Green LED |
D2 | 1 | Red LED |
R9 R10 | 2 | 150 Ω Resistor |
The circuit consists of connecting flex sensors to analog pins (A1 to A5) and servo motors to digital pins (3, 5, 6, 9, 10) on an Arduino board.
The flex sensors act as input devices, measuring the degree of flexion in different parts of the hand.
The Arduino processes these analog signals, mapping them to specific servo motor positions. A lock sensor (pin 2) can be integrated to enable or disable the servo movements based on external input.
Arduino Code:
#include <Servo.h>
const int flexpin1 = A5;
const int flexpin2 = A4;
const int flexpin3 = A3;
const int flexpin4 = A2;
const int flexpin5 = A1;
Servo myServo1;
Servo myServo2;
Servo myServo3;
Servo myServo4;
Servo myServo5;
char lg[100];
int lock = 2;
void setup() {
Serial.begin(9600);
pinMode(lock, INPUT);
myServo1.attach(3);
myServo2.attach(5);
myServo3.attach(6);
myServo4.attach(9);
myServo5.attach(10);
}
void loop() {
int flex1value = analogRead(flexpin1);
int flex2value = analogRead(flexpin2);
int flex3value = analogRead(flexpin3);
int flex4value = analogRead(flexpin4);
int flex5value = analogRead(flexpin5);
int servoPositon1 = map(flex1value, 59, 256, 0, 180);
int servoPositon2 = map(flex2value, 59, 256, 0, 180);
int servoPositon3 = map(flex3value, 59, 256, 0, 180);
int servoPositon4 = map(flex4value, 59, 256, 0, 180);
int servoPositon5 = map(flex5value, 59, 256, 0, 180);
sprintf(lg, "servoPositon1: %d,\tservoPositon2: %d,\tservoPositon3: %d,\tservoPositon4: %d,\tservoPositon5: %d ", servoPositon1, servoPositon2, servoPositon3, servoPositon4, servoPositon5);
Serial.println(lg);
if (digitalRead(lock) == HIGH) {
myServo1.write(servoPositon1);
myServo2.write(servoPositon2);
myServo3.write(servoPositon3);
myServo4.write(servoPositon4);
myServo5.write(servoPositon5);
}
}
This project showcases the potential of integrating flex sensors and servo motors to create an interactive and hands-free control system.
It opens up avenues for applications in prosthetics, robotics, or interactive art, where users can engage with technology using natural hand gestures.
The project not only demonstrates technical proficiency in sensor and servo motor interfacing but also highlights the broader implications of gesture-based control in enhancing human-machine interactions.