LED Electronic Dice Simulator with Arduino
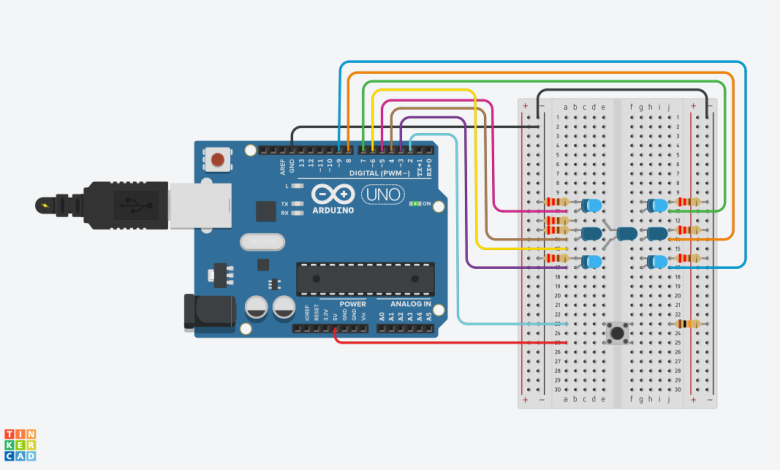
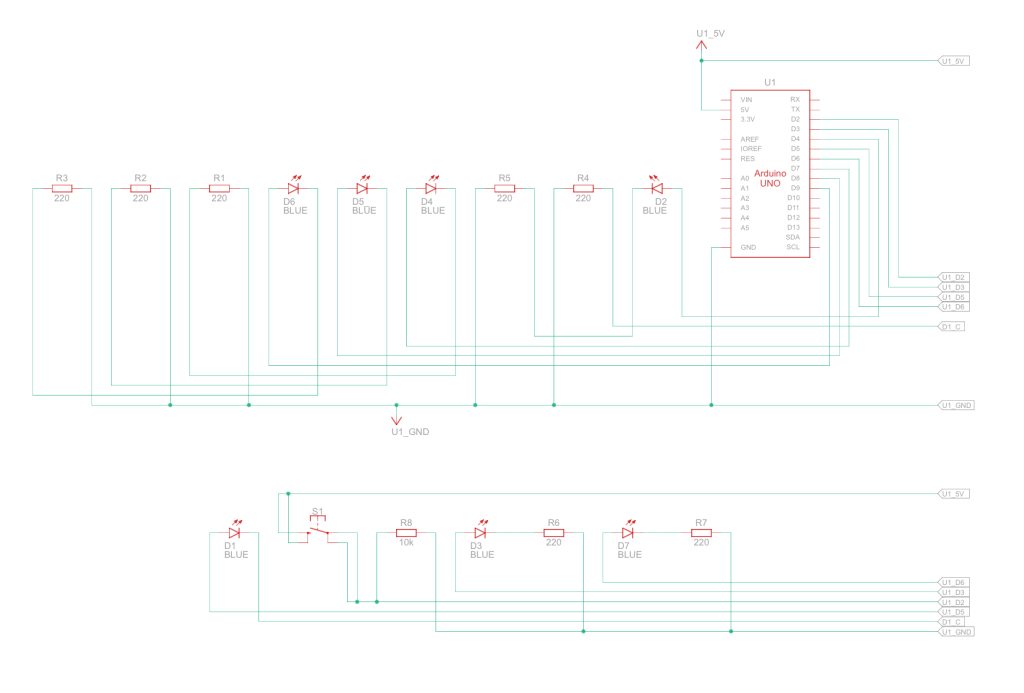
The LED Dice Simulator is an engaging Arduino project that replicates the rolling of a six-sided die using LEDs. It involves an Arduino board connected to buttons and LEDs, creating a simple and interactive dice simulation.
Each LED corresponds to a number on the die, and when a button is pressed, a random LED lights up, mimicking the result of rolling a physical die.
The project enhances both coding and hardware skills, making it suitable for beginners and educational purposes.
Component List:
Name | Quantity | Component |
---|---|---|
U1 | 1 | Arduino Uno R3 |
D1 D2 D3 D4 D5 D6 D7 | 7 | Blue LED |
R1 R2 R3 R4 R5 R6 R7 | 7 | 220 Ω Resistor |
S1 | 1 | Pushbutton |
R8 | 1 | 10 kΩ Resistor |
The circuit consists of an Arduino board connected to eight LEDs (representing the six die faces), and three buttons (simulate the rolling action and initiate the LED display).
Each LED is connected to a digital pin, and the buttons are connected to input pins. This uncomplicated setup allows for easy replication and understanding.
Arduino Code:
The LED Dice Simulator is a fun and educational project for Arduino enthusiasts. It combines programming logic to generate random outcomes with a simple circuit setup, making it an excellent introduction to both software and hardware aspects of Arduino projects. This engaging simulation serves as a hands-on tool for learning about digital inputs, outputs, and the basics of coding, making it a valuable resource for beginners entering the world of Arduino programming.